Java.lang.arrayindexoutofboundsexception: index -1 out of bounds for length 2048
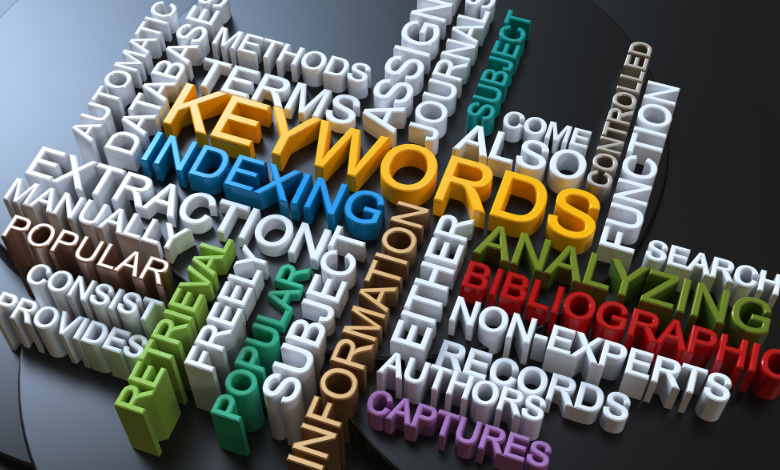
Have you ever encountered the error message, java.Lang.ArrayIndexOutOfBoundsException: index -1 out of bounds for length 2048
, at the same time as operating with Java arrays? If so, you’re now not alone. This is a not unusual exception that builders face, in particular when operating with large datasets or complex algorithms. Understanding why this error occurs and a way to prevent it is crucial for writing sturdy and mistakes-loose code.
Understanding ArrayIndexOutOfBoundsException
Definition of ArrayIndexOutOfBoundsException
The java.Lang.ArrayIndexOutOfBoundsException
is a runtime exception in Java that happens whilst an software attempts to get admission to an array with an invalid index. This can take place if the index is both poor or exceeds the array’s length.
Common Scenarios Where This Exception Occurs
- Accessing elements out of doors the boundaries of an array.
- Iterating via an array with incorrect loop situations.
- Using bad indexes via mistake.
Why the Index -1 Out of Bounds Error?
In Java, array indexing starts at 0. If you attempt to get entry to an array element at index -1, Java will throw an ArrayIndexOutOfBoundsException
, as bad indexes are not legitimate.
How Arrays Work in Java
Structure of Arrays in Java
Arrays in Java are objects that keep a couple of factors of the identical kind. Each detail is accessed thru an index, and those indexes are zero-primarily based, that means the first detail is at index zero.
Zero-Based Indexing in Java
Zero-based totally indexing approach that the primary element of the array is accessed with index 0, the second one element with index 1, and so on. This is a commonplace source of errors for those new to Java or programming in widespread.
How Array Length Is Determined
The length of an array in Java is decided on the time of its creation. Once an array is initialized, its length can not be modified, and any try to get entry to an index outside its bounds will bring about an ArrayIndexOutOfBoundsException
.
Root Causes of the Error
Indexing Beyond the Array Length
One of the maximum common causes of ArrayIndexOutOfBoundsException
is making an attempt to get entry to an index this is more than or same to the duration of the array. For example, if an array has 2048 factors, the valid indexes are 0 to 2047.
Negative Indexes in Arrays
Accessing an array with a negative index, like -1, will without delay throw an ArrayIndexOutOfBoundsException
, as indexes must be non-poor.
Array Initialization Issues
Sometimes, builders may mistakenly assume that an array is properly initialized and try to get admission to its elements without checking its duration, leading to this exception.
Detailed Explanation of “Index -1 Out of Bounds for Length 2048”
What Does the Error Mean?
The mistakes message index -1 out of bounds for length 2048
shows that the program attempted to get entry to an array at index -1, which is not legitimate, for the reason that the array’s valid indexes are from 0 to 2047.
Common Scenarios Leading to the Error
- Subtracting from an index inside a loop without right checks.
- Incorrect calculations that lead to poor indexing.
- Off-by way of-one errors, wherein the loop situation or indexing common sense is inaccurate.
Examples of Code That May Cause This Error
java Copy code int[] myArray = new int[2048]; int index = -1; System.Out.Println(myArray[index]); // This will throw ArrayIndexOutOfBoundsException
In this situation, attempting to get admission to myArray
at index -1 causes the mistake.
Identifying the Error in Your Code
How to Trace the Error in a Large Codebase
When coping with large codebases, locating the source of an ArrayIndexOutOfBoundsException
can be tough. Start via searching on the stack hint, with a view to show you the exact line of code wherein the exception occurred.
Debugging Techniques for IndexOutOfBoundsException
- Use breakpoints to pause execution before the error happens.
- Check array indexes before getting access to elements.
- Print out index values and array lengths for the duration of debugging to make certain they are within valid levels.
Using IDEs to Identify and Fix the Issue
Most present day Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse let you hint and fasten ArrayIndexOutOfBoundsException
via highlighting the elaborate code and suggesting corrections.
Best Practices to Avoid ArrayIndexOutOfBoundsException
Validating Array Indexes Before Access
Always validate that the index you are looking to access is inside the valid variety of the array. This can prevent the exception from going on.
java Copy code if (index >= 0 && index < myArray.Length) System.Out.Println(myArray[index]);
Using Enhanced For-Loop for Safer Iteration
The more suitable for-loop in Java presents a safer manner to iterate over arrays with out stressful about indexes.
java Copy code for (int detail : myArray) System.Out.Println(detail);
Implementing Boundary Checks
Implement assessments that make sure you don’t go out of bounds, specifically when handling dynamic array sizes.
Handling ArrayIndexOutOfBoundsException Gracefully
Try-Catch Blocks to Handle the Exception
Wrap your array get right of entry to code in a try-trap block to deal with the exception gracefully.
java Copy code attempt System.Out.Println(myArray[index]); trap (ArrayIndexOutOfBoundsException e) System.Out.Println("Index out of bounds: " + e.GetMessage());
Logging the Error for Debugging
Log the exception details to assist with debugging, specifically in production environments.
java Copy code catch (ArrayIndexOutOfBoundsException e) logger.Error("Array index out of bounds: " + index, e);
Providing User-Friendly Error Messages
If your software is person-facing, offer a clean and friendly errors message rather of disclosing the exception directly.
Common Mistakes Leading to This Exception
Off-by-One Errors in Loops
These errors arise whilst the loop condition is wrong, leading to an try to get entry to an out-of-bounds index.
java Copy code for (int i = 0; i <= myArray.Period; i++) // Off-via-one error System.Out.Println(myArray[i]);
Incorrectly Calculated Indexes
Ensure that all index calculations are correct and fall inside the array’s bounds.
Misunderstanding Array Length vs. Indexing
Remember that the length of an array isn’t similar to the most index. If an array has a length of 2048, the highest index is 2047.
Preventing Errors Through Testing
Unit Testing for Array Indexes
Create unit assessments that mainly test for boundary situations and make sure that your code doesn’t try to get admission to out-of-bounds indexes.
Automated Testing Tools to Catch the Error
Use computerized testing equipment like JUnit to trap ability ArrayIndexOutOfBoundsException
mistakes earlier than they make it to production.
Code Reviews and Pair Programming
Regular code opinions and pair programming can help trap indexing errors that would cause this exception.
Examples of Correct Array Usage
Safe Accessing of Array Elements
Always make certain that your indexes are within bounds earlier than gaining access to array factors.
java Copy code if (index >= 0 && index < myArray.Length) System.Out.Println(myArray[index]);
Examples with Boundary Checks
java Copy code if (index >= 0 && index < myArray.Length) System.Out.Println(myArray[index]); else System.Out.Println("Index is out of bounds");
Correct Loop Constructs
Make certain your loops do no longer exceed the limits of the array.
java Copy code for (int i = zero; i < myArray.Period; i++) System.Out.Println(myArray[i]);
Advanced Topics
Handling Multi-Dimensional Arrays
Multi-dimensional arrays introduce complexity in indexing. Always make certain that both dimensions are efficaciously indexed.
java Copy code int[][] matrix = new int[10][10]; if (i >= zero && i < matrix.Length && j >= 0 && j < matrix[i].Length) System.Out.Println(matrix[i][j]);
Working with ArrayLists and Other Collections
ArrayList
and different collections in Java handle bounds internally, however you should nevertheless be careful with index-based totally get entry to.
Using Custom Exceptions for Better Error Handling
Consider growing custom exceptions to provide more context when array bounds are surpassed.
Optimizing Performance with Large Arrays
Memory Management with Large Arrays
When dealing with huge arrays, consider of reminiscence intake. Ensure that your application can deal with the array size with out running into reminiscence issues.
Efficient Index Calculations
Optimize your index calculations to avoid needless computations that might slow down your application.
Best Practices for Working with Large Datasets
Consider the use of information systems extra suited for large datasets, together with ArrayList
, that may grow dynamically.
FAQs about ArrayIndexOutOfBoundsException
What is the distinction between ArrayIndexOutOfBoundsException and IndexOutOfBoundsException?
ArrayIndexOutOfBoundsException
is precise to arrays, at the same time as IndexOutOfBoundsException
can arise with different information structures like lists.
How can I avoid poor index mistakes in Java?
Always validate your indexes earlier than using them to get admission to array factors.
What gear can I use to debug IndexOutOfBoundsException?
Use IDEs like IntelliJ IDEA or Eclipse, which offer debugging gear which could assist hint and fasten this exception.
How does array initialization have an effect on indexing?
Improper initialization can cause surprising behavior, so constantly make certain that arrays are nicely initialized before use.
Can this exception arise with ArrayLists in Java?
Yes, however ArrayList
normally manages bounds internally, so this exception is less commonplace until explicitly misused.
Conclusion
Understanding and handling java.Lang.ArrayIndexOutOfBoundsException: index -1 out of bounds for length 2048
is important for any Java developer. By following great practices, acting thorough checking out, and using debugging gear, you could save you this commonplace errors from disrupting your code. Always keep in mind to validate your array indexes and take care of exceptions gracefully to create sturdy and reliable applications.